AMP-MMV Demo
This demo file will provide several examples of how to use the various tools that make up the AMP-MMV software package. We realize that there is a lot to read in this demo, but taking the time to carefully follow the examples we've provided should provide the reader with a fairly thorough understanding of how to correctly operate AMP-MMV. The file tutorial.pdf in the top-level directory of this software package provides a more visually appealing version of this demo.
Note that in order for this demo script to work properly, all of the folders in the AMP-MMV software package must be added to MATLAB's path. This can be accomplished easily by running the script add2path.m, which can be found in the top-level directory of this software package.
Contents
Coded by: Justin Ziniel, The Ohio State Univ. E-mail: zinielj @ ece.osu.edu Last change: 12/29/11 Change summary: - Created from demo v1.0 (12/29/11; JAZ) Version 1.1
% Initialize the random number stream RandStream.setDefaultStream(RandStream('mt19937ar', 'Seed', 0));
Example 1: A Simple Recovery
In this first example, we will demonstrate how to obtain a sample MMV recovery with just a few lines of code.
First, let's generate some synthetic data according to AMP-MMV's signal model. To do this, we will use the function SIGNAL_GEN_FXN, which requires as an input an object of the custom-defined class SigGenParams. (The class definition file can be found in the folder ClassDefs.) There are several ways to create objects of this class. The easiest is to use a constructor with no arguments, which will construct an object using all default values for the parameters of the signal model.
SigGenObj = SigGenParams(); % Call the default constructor
Now we can look at the parameter values associated with our object by using its print method, which will print the current configuration to the command window.
SigGenObj.print(); % Print the current signal model configuration
********************************************* Signal Generation Parameters ********************************************* Signal dimension (N): 1024 Measurement dimension (M): 256 # of timesteps (T): 4 Measurement matrix type: IID Gaussian Activity probability (lambda): 0.08 Active mean (zeta): 0 Active variance (sigma2): 1 Innovation rate (alpha): 0.1 Empirical SNR (SNRmdB): 25 dB Data type: Complex-valued
By looking at the command window printout, we can see that the default signal model consists of a signal of length N = 1024, measurement vectors of length M = 256, and a total of T = 4 timesteps. The measurement matrix will be randomly constructed with IID Gaussian elements. The next several parameters specify the signal prior. We see that the apriori probability that a given coefficient x_n(t) is non-zero is given by lambda = 0.08. For those coefficients that are non-zero, they have a mean of zeta = 0, and a variance of sigma2 = 1. The active coefficient amplitudes evolve over time with a correlation equal to 1 - alpha = 1 - 0.10 = 0.90. Finally, we see that the additive noise will have a variance chosen to yield an SNR of 25dB, and that the data generated will be complex-valued.
Having constructed the object that contains our model parameters, we can now pass it to SIGNAL_GEN_FXN, which will produce 6 outputs...
[x_true, y, A, support, K, sig2e] = signal_gen_fxn(SigGenObj);
...the first of which, x_true, is a 1-by-T cell array, with cell t containing the value of the true length-N signal at timestep t, i.e., x_true{t}. The second output, y, is a 1-by-T cell array of length-M measurements. The third output, A, is a 1-by-T cell array of M-by-N (identical) measurement matrices. (We defer for now the reason for giving duplicates of the measurement matrix.) The fourth output is a vector of indices of the non-zero coefficients of the true signal, and the fifth output, K, is the cardinality of the support (i.e., the number of true non-zeroes). The sixth and final output, sig2e, is the variance of the AWGN corrupting noise.
Now that we have a realization to work with, we can now use AMP-MMV to attempt to recover x_true from the noisy measurements, y. The easiest way to do this is to call SP_MMV_WRAPPER_FXN, which will try several different configurations of AMP-MMV (e.g., different message passing schedules, different numbers of smoothing iterations, etc.) until it finds a solution with a residual energy that is small enough.
In order to use SP_MMV_WRAPPER_FXN, we need at least y, A, and a third input, Params, which is an object of the class ModelParams, and contains parameters that specify the signal model. Since we have generated data according to our signal model, (and for now, we will assume perfect knowledge of the model parameters), we can construct a Params object easily by passing our SigGenParams object, SigGenObj, to the ModelParams constructor, along with sig2e, and it will copy over the required parameters:
Params = ModelParams(SigGenObj, sig2e); % Construct ModelParams object
Now let's verify that our Params object was constructed correctly by printing its property values to the command window:
Params.print(); % Print the value of the Params object properties
**************************************** Signal Model Parameters **************************************** Activity probability (lambda): 0.08 Active mean (zeta): 0 Active variance (sigma2): 1 Innovation rate (alpha): 0.1 AWGN variance (sig2e): 0.00092579
Everything looks good here, so we are now ready to call SP_MMV_WRAPPER_FXN. Since we are not providing an optional fourth input of runtime configuration options, AMP-MMV will use a default runtime configuration.
% Obtain a recovery using AMP-MMV
[x_hat, v_hat, lambda_hat] = sp_mmv_wrapper_fxn(y, A, Params);
*********************************** AMP-MMV Runtime Options *********************************** Max. smoothing iterations: 5 Min. smoothing iterations: 5 Max. AMP/BP iterations: 15 Intra-frame algorithm: AMP EM parameter learning: Yes Update groups: [Default] Verbosity: Verbose Warm-Start: No epsilon: 1e-06 f-to-theta approx (tau): Taylor series approx ************************************************************************************************* Running parallel schedule w/ Taylor approx. & smooth_iters = 5, min_iters = 5, inner_iters = 15 SP_MMV_FXN: Completed 1 iterations Total elapsed time: 0.039207 s Time-averaged residual energy: 0.166055 --------------------------------------------------------- SP_MMV_FXN: Completed 2 iterations Total elapsed time: 0.053362 s Time-averaged residual energy: 0.173272 --------------------------------------------------------- SP_MMV_FXN: Completed 3 iterations Total elapsed time: 0.067525 s Time-averaged residual energy: 0.173192 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.080000 Updated value of 'alpha' for Group 1: 0.100000 Updated value of 'zeta' for Group 1: 0.0022397-0.0055706i Updated value of 'sigma2' for Group 1: 0.975009 Updated value of 'sig2e': 0.001007 --------------------------------------------------------- SP_MMV_FXN: Completed 4 iterations Total elapsed time: 0.093063 s Time-averaged residual energy: 0.173271 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.066407 Updated value of 'alpha' for Group 1: 0.099598 Updated value of 'zeta' for Group 1: 0.0022397-0.0055706i Updated value of 'sigma2' for Group 1: 0.970842 Updated value of 'sig2e': 0.001034 --------------------------------------------------------- SP_MMV_FXN: Completed 5 iterations Total elapsed time: 0.114810 s Time-averaged residual energy: 0.173335 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.066407 Updated value of 'alpha' for Group 1: 0.099598 Updated value of 'zeta' for Group 1: 0.0042776-0.010685i Updated value of 'sigma2' for Group 1: 0.963186 Updated value of 'sig2e': 0.001045 --------------------------------------------------------- sp_mmv_fxn: Max # of smoothing iterations reached Residual energy ratio (actual/expected): 0.731362 Returning recovery from parallel schedule w/ Taylor approx. & smooth_iters = 5, min_iters = 5, inner_iters = 15 *************************************************************************************************
The first output, x_hat, is a 1-by-T cell array of MMSE signal estimates. The second output, v_hat, contains the posterior marginal variances of each MMSE estimate in a 1-by-T cell array, i.e., v_hat{t}(n) = var{x_n(t) | y}. The final output, |lambda_hat, is a length-N vector of posterior activity probabilities, i.e., lambda_hat(n) = p(s_n = 1 | y).
In addition to returning these three outputs, SP_MMV_WRAPPER_FXN has printed some information to the command window. We see that the first things that it printed were the runtime options that it was using (the defaults) during execution. For example, we can see from the printout that the maximum number of smoothing iterations equaled the minimum number (5), and that during the AMP message passes that occurred within each frame, a maximum of 15 AMP iterations were allowed. The EM parameter learning procedure is enabled by default, and the algorithm is set to operate verbosely. We also see that it first attempted a parallel message passing schedule, and we can observe the EM parameter updates of each of the model parameters after each smoothing iteration. Since the residual energy obtained from using this initial schedule was below a pre-defined threshold, the algorithm terminates.
Now let's check the quality of the solution obtained using AMP-MMV. We'll use timestep-averaged normalized mean square error (TNMSE) and the normalized support error rate (NSER) as our performance metrics. As a support estimate, we will use a simple ML estimator that relies on our vector of posterior activity probabilities, lambda_hat.
s_hat = (lambda_hat > 1/2); % ML support estimate T = SigGenObj.T; % Total # of timesteps TNMSE = sum(sum(([x_true{:}]-[x_hat{:}]).^2, 1)./sum([x_true{:}].^2, 1))/T; NSER = nser(support, find(s_hat == 1)); fprintf('TNMSE: %g dB\n', 10*log10(TNMSE)); fprintf('NSER: %g\n', NSER);
TNMSE: -25.6568 dB NSER: 0
From the printouts to the command window, we see that AMP-MMV performed well on this problem in both TNMSE and NSER. To finish this example, let's visually observe the true signal and the recovered signal:
figure(1); subplot(121); imagesc(abs([x_true{:}])); colorbar xlabel('Timestep (t)'); ylabel('Index [n]'); title('True signal') subplot(122); imagesc(abs([x_hat{:}])); colorbar xlabel('Timestep (t)'); ylabel('Index [n]'); title('AMP-MMV recovery')
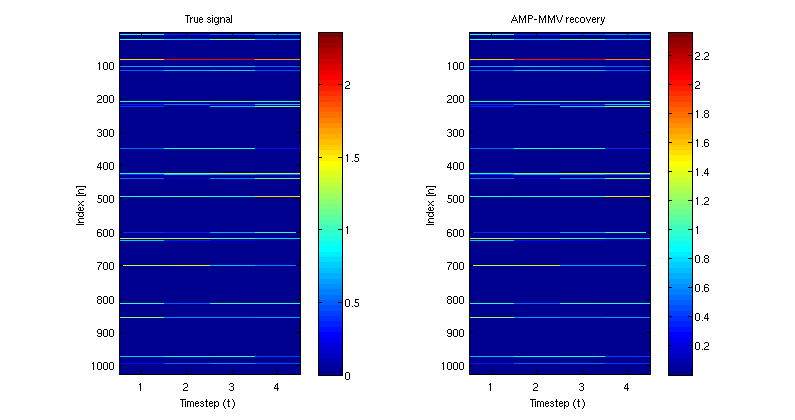
Example 2: Customizing AMP-MMV
In the previous example, we used default values for all of the functions that we called. In this example, we will illustrate how to choose non-default values for the parameters.
As before, we will generate synthetic data according to our signal model by calling SIGNAL_GEN_FXN. Once again, we start by constructing an object of the SigGenParams class. Previously, we called the SigGenParams constructor with no arguments, which created an object of all default values. Suppose now that we'd like to come up with a more challenging test case. Let's keep the signal dimension, N, the same, but reduce the number of measurements at each timestep, M, to 128, giving an undersampling ratio of N/M = 8. Let's likewise adjust the prior activity probability, lambda, so that M/K = 2, that is, lambda = 0.0625. We can use the following constructor call:
SigGenObj = SigGenParams('M', 128, 'lambda', 0.0625); SigGenObj.print();
********************************************* Signal Generation Parameters ********************************************* Signal dimension (N): 1024 Measurement dimension (M): 128 # of timesteps (T): 4 Measurement matrix type: IID Gaussian Activity probability (lambda): 0.0625 Active mean (zeta): 0 Active variance (sigma2): 1 Innovation rate (alpha): 0.1 Empirical SNR (SNRmdB): 25 dB Data type: Complex-valued
From the printout to the command window, we can see that SigGenObj has used our two custom settings, and left everything else at their default values. Suppose we realize now that we wanted to change the innovation rate, alpha, as well, to alpha = 0.05. We could just construct SigGenObj all over again, and include our custom choice of alpha in the constructor call. Alternatively, we can change the value of alpha for the object we just constructed:
SigGenObj.alpha = 0.05; SigGenObj.print();
********************************************* Signal Generation Parameters ********************************************* Signal dimension (N): 1024 Measurement dimension (M): 128 # of timesteps (T): 4 Measurement matrix type: IID Gaussian Activity probability (lambda): 0.0625 Active mean (zeta): 0 Active variance (sigma2): 1 Innovation rate (alpha): 0.05 Empirical SNR (SNRmdB): 25 dB Data type: Complex-valued
From the printout, we see that the value of alpha was successfully updated.
Now, let's generate our synthetic data by calling SIGNAL_GEN_FXN:
[x_true, y, A, support, K, sig2e] = signal_gen_fxn(SigGenObj);
As before, in order to obtain a recovery with AMP-MMV, we need to provide it with an initialization of the model parameters. Last time, we created a ModelParams object, Params, with the true parameter values as an initialization by using the constructor ModelParams(SigGenParams, sig2e). This time, let's start with an initialization that is a little bit off from the true values. To do this, we will use an alternative constructor, which has the syntax ModelParams(lambda, zeta, sigma2, alpha, sig2e). Here's how we'll initialize:
Params = ModelParams((1/4)*SigGenObj.lambda, 0, 2, 0.029, 1/2*sig2e); Params.print();
**************************************** Signal Model Parameters **************************************** Activity probability (lambda): 0.015625 Active mean (zeta): 0 Active variance (sigma2): 2 Innovation rate (alpha): 0.029 AWGN variance (sig2e): 0.00068928
In the last example, we used default runtime options for AMP-MMV. This time, let's customize some of those options. To do this, we need to create an object of the class Options, which we will then pass to AMP-MMV. Since this is a more challenging example, let's increase the default maximum number of smoothing iterations to 10, and let's increase the maximum number of inner AMP iterations to 25. We can use the following constructor:
RunOpt = Options('smooth_iters', 10, 'inner_iters', 25); RunOpt.print();
*********************************** AMP-MMV Runtime Options *********************************** Max. smoothing iterations: 10 Min. smoothing iterations: 5 Max. AMP/BP iterations: 25 Intra-frame algorithm: AMP EM parameter learning: Yes Update groups: [Default] Verbosity: Silent Warm-Start: No epsilon: 1e-06 f-to-theta approx (tau): Taylor series approx
As we can see from the command window printout, our custom options appear within the RunOpt object we just created. Notice though that our constructed object has verbosity defaulting to silent. Let's change this so that we can see what AMP-MMV is doing while it runs.
RunOpt.verbose = true; RunOpt.print();
*********************************** AMP-MMV Runtime Options *********************************** Max. smoothing iterations: 10 Min. smoothing iterations: 5 Max. AMP/BP iterations: 25 Intra-frame algorithm: AMP EM parameter learning: Yes Update groups: [Default] Verbosity: Verbose Warm-Start: No epsilon: 1e-06 f-to-theta approx (tau): Taylor series approx
Now everything is ready for us to call AMP-MMV. As before, we'll use SP_MMV_WRAPPER_FXN to allow us to try multiple different schedules, only this time we will provide it with our custom runtime options.
[x_hat, v_hat, lambda_hat] = sp_mmv_wrapper_fxn(y, A, Params, RunOpt);
************************************************************************************************* Running parallel schedule w/ Taylor approx. & smooth_iters = 10, min_iters = 5, inner_iters = 25 SP_MMV_FXN: Completed 1 iterations Total elapsed time: 0.058439 s Time-averaged residual energy: 2.432096 --------------------------------------------------------- SP_MMV_FXN: Completed 2 iterations Total elapsed time: 0.078901 s Time-averaged residual energy: 0.100955 --------------------------------------------------------- SP_MMV_FXN: Completed 3 iterations Total elapsed time: 0.093257 s Time-averaged residual energy: 0.087364 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.015625 Updated value of 'alpha' for Group 1: 0.029000 Updated value of 'zeta' for Group 1: 0.0014796-0.0084173i Updated value of 'sigma2' for Group 1: 1.720171 Updated value of 'sig2e': 0.001307 --------------------------------------------------------- SP_MMV_FXN: Completed 4 iterations Total elapsed time: 0.110889 s Time-averaged residual energy: 0.096756 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.053880 Updated value of 'alpha' for Group 1: 0.028812 Updated value of 'zeta' for Group 1: 0.0014796-0.0084173i Updated value of 'sigma2' for Group 1: 1.708716 Updated value of 'sig2e': 0.001733 --------------------------------------------------------- SP_MMV_FXN: Completed 5 iterations Total elapsed time: 0.127429 s Time-averaged residual energy: 0.105677 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.053880 Updated value of 'alpha' for Group 1: 0.028812 Updated value of 'zeta' for Group 1: 0.0025874-0.015758i Updated value of 'sigma2' for Group 1: 1.675061 Updated value of 'sig2e': 0.002009 --------------------------------------------------------- SP_MMV_FXN: Completed 6 iterations Total elapsed time: 0.143734 s Time-averaged residual energy: 0.106906 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.053733 Updated value of 'alpha' for Group 1: 0.028761 Updated value of 'zeta' for Group 1: 0.0025874-0.015758i Updated value of 'sigma2' for Group 1: 1.672036 Updated value of 'sig2e': 0.002201 --------------------------------------------------------- SP_MMV_FXN: Completed 7 iterations Total elapsed time: 0.159999 s Time-averaged residual energy: 0.108514 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.053733 Updated value of 'alpha' for Group 1: 0.028761 Updated value of 'zeta' for Group 1: 0.0038061-0.022855i Updated value of 'sigma2' for Group 1: 1.662872 Updated value of 'sig2e': 0.002337 --------------------------------------------------------- SP_MMV_FXN: Completed 8 iterations Total elapsed time: 0.176552 s Time-averaged residual energy: 0.109349 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.053716 Updated value of 'alpha' for Group 1: 0.028747 Updated value of 'zeta' for Group 1: 0.0038061-0.022855i Updated value of 'sigma2' for Group 1: 1.662066 Updated value of 'sig2e': 0.002434 --------------------------------------------------------- SP_MMV_FXN: Completed 9 iterations Total elapsed time: 0.192742 s Time-averaged residual energy: 0.110013 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.053716 Updated value of 'alpha' for Group 1: 0.028747 Updated value of 'zeta' for Group 1: 0.0049578-0.029565i Updated value of 'sigma2' for Group 1: 1.655693 Updated value of 'sig2e': 0.002505 --------------------------------------------------------- SP_MMV_FXN: Completed 10 iterations Total elapsed time: 0.209157 s Time-averaged residual energy: 0.110454 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.053713 Updated value of 'alpha' for Group 1: 0.028743 Updated value of 'zeta' for Group 1: 0.0049578-0.029565i Updated value of 'sigma2' for Group 1: 1.655443 Updated value of 'sig2e': 0.002555 --------------------------------------------------------- sp_mmv_fxn: Max # of smoothing iterations reached Residual energy ratio (actual/expected): 1.25191 Running serial schedule w/ Taylor approx. & smooth_iters = 10, min_iters = 5, inner_iters = 25 SP_MMV_SERIAL_FXN: Completed 1 forward and 0 backward iterations Total elapsed time: 0.011642 s Time-averaged residual energy: 0.101638 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 1 forward and 1 backward iterations Total elapsed time: 0.020532 s Time-averaged residual energy: 0.095457 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 2 forward and 1 backward iterations Total elapsed time: 0.032144 s Time-averaged residual energy: 0.090395 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 2 forward and 2 backward iterations Total elapsed time: 0.040922 s Time-averaged residual energy: 0.084839 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 3 forward and 2 backward iterations Total elapsed time: 0.052429 s Time-averaged residual energy: 0.078083 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 3 forward and 3 backward iterations Total elapsed time: 0.061303 s Time-averaged residual energy: 0.079161 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.015625 Updated value of 'alpha' for Group 1: 0.029000 Updated value of 'zeta' for Group 1: 0.0058069-0.035203i Updated steady-state variance for Group 1: 1.873277 Updated value of 'sig2e': 0.001286 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 4 forward and 3 backward iterations Total elapsed time: 0.078746 s Time-averaged residual energy: 0.096478 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 4 forward and 4 backward iterations Total elapsed time: 0.087426 s Time-averaged residual energy: 0.101428 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.054064 Updated value of 'alpha' for Group 1: 0.028511 Updated value of 'zeta' for Group 1: 0.0058069-0.035203i Updated steady-state variance for Group 1: 1.840908 Updated value of 'sig2e': 0.001756 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 5 forward and 4 backward iterations Total elapsed time: 0.102884 s Time-averaged residual energy: 0.103547 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 5 forward and 5 backward iterations Total elapsed time: 0.111523 s Time-averaged residual energy: 0.105613 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.054064 Updated value of 'alpha' for Group 1: 0.028511 Updated value of 'zeta' for Group 1: 0.0068213-0.041078i Updated steady-state variance for Group 1: 1.783917 Updated value of 'sig2e': 0.002046 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 6 forward and 5 backward iterations Total elapsed time: 0.127162 s Time-averaged residual energy: 0.106937 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 6 forward and 6 backward iterations Total elapsed time: 0.135879 s Time-averaged residual energy: 0.107282 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.053720 Updated value of 'alpha' for Group 1: 0.028349 Updated value of 'zeta' for Group 1: 0.0068213-0.041078i Updated steady-state variance for Group 1: 1.773553 Updated value of 'sig2e': 0.002245 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 7 forward and 6 backward iterations Total elapsed time: 0.151515 s Time-averaged residual energy: 0.108250 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 7 forward and 7 backward iterations Total elapsed time: 0.160270 s Time-averaged residual energy: 0.108402 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.053720 Updated value of 'alpha' for Group 1: 0.028349 Updated value of 'zeta' for Group 1: 0.0078092-0.046801i Updated steady-state variance for Group 1: 1.761148 Updated value of 'sig2e': 0.002382 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 8 forward and 7 backward iterations Total elapsed time: 0.176106 s Time-averaged residual energy: 0.109112 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 8 forward and 8 backward iterations Total elapsed time: 0.184687 s Time-averaged residual energy: 0.109211 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.053713 Updated value of 'alpha' for Group 1: 0.028287 Updated value of 'zeta' for Group 1: 0.0078092-0.046801i Updated steady-state variance for Group 1: 1.757162 Updated value of 'sig2e': 0.002478 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 9 forward and 8 backward iterations Total elapsed time: 0.200311 s Time-averaged residual energy: 0.109722 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 9 forward and 9 backward iterations Total elapsed time: 0.208967 s Time-averaged residual energy: 0.109788 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.053713 Updated value of 'alpha' for Group 1: 0.028287 Updated value of 'zeta' for Group 1: 0.0087583-0.052284i Updated steady-state variance for Group 1: 1.747922 Updated value of 'sig2e': 0.002543 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 10 forward and 9 backward iterations Total elapsed time: 0.224939 s Time-averaged residual energy: 0.110164 --------------------------------------------------------- SP_MMV_SERIAL_FXN: Completed 10 forward and 10 backward iterations Total elapsed time: 0.233481 s Time-averaged residual energy: 0.110226 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.053711 Updated value of 'alpha' for Group 1: 0.028258 Updated value of 'zeta' for Group 1: 0.0087583-0.052284i Updated steady-state variance for Group 1: 1.746129 Updated value of 'sig2e': 0.002588 --------------------------------------------------------- sp_mmv_serial_fxn: Max # of smoothing iterations reached Residual energy ratio (actual/expected): 1.24933 Returning recovery from serial schedule w/ Taylor approx. & smooth_iters = 10, min_iters = 5, inner_iters = 25 *************************************************************************************************
Once again, we see that SP_MMV_WRAPPER_FXN first tried a parallel message passing schedule, however this time, the resultant residual energy was not low enough, given our initialization of sig2e, to allow AMP-MMV to terminate. Therefore, it next attempted a serial message passing schedule, also with 10 smoothing iterations. This time, the residual energy is low enough, and so SP_MMV_WRAPPER_FXN reports that it is returning the recovery from the serial message passing schedule.
Once more, let's compute our performance metrics, and look at a plot of our recovery:
s_hat = (lambda_hat > 1/2); TNMSE = sum(sum(([x_true{:}]-[x_hat{:}]).^2, 1)./sum([x_true{:}].^2, 1))/T; NSER = nser(support, find(s_hat == 1)); fprintf('TNMSE: %g dB\n', 10*log10(TNMSE)); fprintf('NSER: %g\n', NSER); figure(1); subplot(121); imagesc(abs([x_true{:}])); colorbar xlabel('Timestep (t)'); ylabel('Index [n]'); title('True signal') subplot(122); imagesc(abs([x_hat{:}])); colorbar xlabel('Timestep (t)'); ylabel('Index [n]'); title('AMP-MMV recovery')
TNMSE: -35.1386 dB NSER: 0
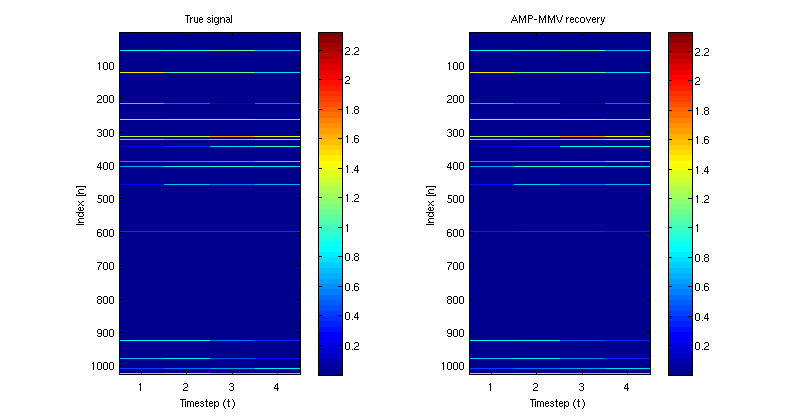
With a TNMSE of -35 dB, and an NSER of 0, we can see that AMP-MMV performed quite well, despite the fact that we initialized it with inaccurate model parameters, which it refined during execution.
Example 3: Using a particular schedule, and running the SKS
In previous experiments, we used SP_MMV_WRAPPER_FXN to allow us to cycle through multiple different configurations of AMP-MMV if needed, changing the schedule between serial and parallel, and adjusting the number of smoothing iterations. In this example, we will illustrate how to obtain a recovery using AMP-MMV only once, by calling the function that implements the specific message passing schedule we wish to use. We will also demonstrate how to obtain a lower bound on the achievable TNMSE performance by running the support-aware Kalman smoother.
If we are only interested in the parallel message passing schedule, then we can call the function SP_MMV_FXN, which accepts the same arguments as SP_MMV_WRAPPER_FXN. Alternatively, if we are interested in the serial message passing schedule, which likewise uses the same arguments as SP_MMV_WRAPPER_FXN, then the appropriate function call is SP_MMV_SERIAL_FXN.
For this example, let's use the parallel message passing schedule. First let's generate a signal according to our signal model, with some customizations of the default signal model. Let's also try generating real-valued, rather than complex-valued data
SigGenObj = SigGenParams('M', 160, 'lambda', 0.0625, 'zeta', 1, ... 'version', 'R'); SigGenObj.print(); [x_true, y, A, support, K, sig2e] = signal_gen_fxn(SigGenObj);
********************************************* Signal Generation Parameters ********************************************* Signal dimension (N): 1024 Measurement dimension (M): 160 # of timesteps (T): 4 Measurement matrix type: IID Gaussian Activity probability (lambda): 0.0625 Active mean (zeta): 1 Active variance (sigma2): 1 Innovation rate (alpha): 0.1 Empirical SNR (SNRmdB): 25 dB Data type: Real-valued
Now we need to create our ModelParams object. Let's provide AMP-MMV with an inaccurate initialization once again. Let's also create a custom Options object with an increased number of smoothing iterations. We'll bump this maximum number of smoothing iterations way up so that we can observe the parameter estimates move towards their true values.
Params = ModelParams(1/5*SigGenObj.lambda, 0, 1, 0.50, 1e-3); Params.print(); RunOpt = Options('smooth_iters', 70, 'verbose', true); RunOpt.print();
**************************************** Signal Model Parameters **************************************** Activity probability (lambda): 0.0125 Active mean (zeta): 0 Active variance (sigma2): 1 Innovation rate (alpha): 0.5 AWGN variance (sig2e): 0.001 *********************************** AMP-MMV Runtime Options *********************************** Max. smoothing iterations: 70 Min. smoothing iterations: 5 Max. AMP/BP iterations: 15 Intra-frame algorithm: AMP EM parameter learning: Yes Update groups: [Default] Verbosity: Verbose Warm-Start: No epsilon: 1e-06 f-to-theta approx (tau): Taylor series approx
Now we are ready to directly call the parallel message passing schedule implementation of AMP-MMV:
[x_hat, v_hat, lambda_hat] = sp_mmv_fxn(y, A, Params, RunOpt);
SP_MMV_FXN: Completed 1 iterations Total elapsed time: 0.033556 s Time-averaged residual energy: 37.571589 --------------------------------------------------------- SP_MMV_FXN: Completed 2 iterations Total elapsed time: 0.067204 s Time-averaged residual energy: 3.251477 --------------------------------------------------------- SP_MMV_FXN: Completed 3 iterations Total elapsed time: 0.100598 s Time-averaged residual energy: 25.175767 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.012500 Updated value of 'alpha' for Group 1: 0.500000 Updated value of 'zeta' for Group 1: 0.035917 Updated value of 'sigma2' for Group 1: 0.871366 Updated value of 'sig2e': 0.211323 --------------------------------------------------------- SP_MMV_FXN: Completed 4 iterations Total elapsed time: 0.131533 s Time-averaged residual energy: 3.457915 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.024905 Updated value of 'alpha' for Group 1: 0.482918 Updated value of 'zeta' for Group 1: 0.035917 Updated value of 'sigma2' for Group 1: 0.831727 Updated value of 'sig2e': 0.255929 --------------------------------------------------------- SP_MMV_FXN: Completed 5 iterations Total elapsed time: 0.153431 s Time-averaged residual energy: 20.038591 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.024905 Updated value of 'alpha' for Group 1: 0.482918 Updated value of 'zeta' for Group 1: 0.071914 Updated value of 'sigma2' for Group 1: 0.843570 Updated value of 'sig2e': 0.192565 --------------------------------------------------------- SP_MMV_FXN: Completed 6 iterations Total elapsed time: 0.171749 s Time-averaged residual energy: 8.822881 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.034923 Updated value of 'alpha' for Group 1: 0.474286 Updated value of 'zeta' for Group 1: 0.071914 Updated value of 'sigma2' for Group 1: 0.823606 Updated value of 'sig2e': 0.134117 --------------------------------------------------------- SP_MMV_FXN: Completed 7 iterations Total elapsed time: 0.189825 s Time-averaged residual energy: 9.096523 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.034923 Updated value of 'alpha' for Group 1: 0.474286 Updated value of 'zeta' for Group 1: 0.10362 Updated value of 'sigma2' for Group 1: 0.814196 Updated value of 'sig2e': 0.120013 --------------------------------------------------------- SP_MMV_FXN: Completed 8 iterations Total elapsed time: 0.207346 s Time-averaged residual energy: 5.775555 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.041775 Updated value of 'alpha' for Group 1: 0.462948 Updated value of 'zeta' for Group 1: 0.10362 Updated value of 'sigma2' for Group 1: 0.788617 Updated value of 'sig2e': 0.099559 --------------------------------------------------------- SP_MMV_FXN: Completed 9 iterations Total elapsed time: 0.225194 s Time-averaged residual energy: 6.404340 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.041775 Updated value of 'alpha' for Group 1: 0.462948 Updated value of 'zeta' for Group 1: 0.12925 Updated value of 'sigma2' for Group 1: 0.769712 Updated value of 'sig2e': 0.095520 --------------------------------------------------------- SP_MMV_FXN: Completed 10 iterations Total elapsed time: 0.244246 s Time-averaged residual energy: 4.631725 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.046216 Updated value of 'alpha' for Group 1: 0.450849 Updated value of 'zeta' for Group 1: 0.12925 Updated value of 'sigma2' for Group 1: 0.743481 Updated value of 'sig2e': 0.086856 --------------------------------------------------------- SP_MMV_FXN: Completed 11 iterations Total elapsed time: 0.261461 s Time-averaged residual energy: 5.246462 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.046216 Updated value of 'alpha' for Group 1: 0.450849 Updated value of 'zeta' for Group 1: 0.1519 Updated value of 'sigma2' for Group 1: 0.721696 Updated value of 'sig2e': 0.083801 --------------------------------------------------------- SP_MMV_FXN: Completed 12 iterations Total elapsed time: 0.278737 s Time-averaged residual energy: 4.065438 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.051105 Updated value of 'alpha' for Group 1: 0.438995 Updated value of 'zeta' for Group 1: 0.1519 Updated value of 'sigma2' for Group 1: 0.697140 Updated value of 'sig2e': 0.076595 --------------------------------------------------------- SP_MMV_FXN: Completed 13 iterations Total elapsed time: 0.295297 s Time-averaged residual energy: 4.289547 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.051105 Updated value of 'alpha' for Group 1: 0.438995 Updated value of 'zeta' for Group 1: 0.17221 Updated value of 'sigma2' for Group 1: 0.675453 Updated value of 'sig2e': 0.071838 --------------------------------------------------------- SP_MMV_FXN: Completed 14 iterations Total elapsed time: 0.311357 s Time-averaged residual energy: 3.497187 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.055785 Updated value of 'alpha' for Group 1: 0.427594 Updated value of 'zeta' for Group 1: 0.17221 Updated value of 'sigma2' for Group 1: 0.652917 Updated value of 'sig2e': 0.066238 --------------------------------------------------------- SP_MMV_FXN: Completed 15 iterations Total elapsed time: 0.326734 s Time-averaged residual energy: 3.438987 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.055785 Updated value of 'alpha' for Group 1: 0.427594 Updated value of 'zeta' for Group 1: 0.19051 Updated value of 'sigma2' for Group 1: 0.630706 Updated value of 'sig2e': 0.061069 --------------------------------------------------------- SP_MMV_FXN: Completed 16 iterations Total elapsed time: 0.341628 s Time-averaged residual energy: 3.037180 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.059301 Updated value of 'alpha' for Group 1: 0.416331 Updated value of 'zeta' for Group 1: 0.19051 Updated value of 'sigma2' for Group 1: 0.609516 Updated value of 'sig2e': 0.055596 --------------------------------------------------------- SP_MMV_FXN: Completed 17 iterations Total elapsed time: 0.356126 s Time-averaged residual energy: 2.919550 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.059301 Updated value of 'alpha' for Group 1: 0.416331 Updated value of 'zeta' for Group 1: 0.2054 Updated value of 'sigma2' for Group 1: 0.586927 Updated value of 'sig2e': 0.051224 --------------------------------------------------------- SP_MMV_FXN: Completed 18 iterations Total elapsed time: 0.369315 s Time-averaged residual energy: 2.727802 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.061478 Updated value of 'alpha' for Group 1: 0.405275 Updated value of 'zeta' for Group 1: 0.2054 Updated value of 'sigma2' for Group 1: 0.567183 Updated value of 'sig2e': 0.048383 --------------------------------------------------------- SP_MMV_FXN: Completed 19 iterations Total elapsed time: 0.381845 s Time-averaged residual energy: 2.603785 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.061478 Updated value of 'alpha' for Group 1: 0.405275 Updated value of 'zeta' for Group 1: 0.21619 Updated value of 'sigma2' for Group 1: 0.545471 Updated value of 'sig2e': 0.046867 --------------------------------------------------------- SP_MMV_FXN: Completed 20 iterations Total elapsed time: 0.395462 s Time-averaged residual energy: 2.454229 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.063323 Updated value of 'alpha' for Group 1: 0.394644 Updated value of 'zeta' for Group 1: 0.21619 Updated value of 'sigma2' for Group 1: 0.527466 Updated value of 'sig2e': 0.046019 --------------------------------------------------------- SP_MMV_FXN: Completed 21 iterations Total elapsed time: 0.408845 s Time-averaged residual energy: 2.352970 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.063323 Updated value of 'alpha' for Group 1: 0.394644 Updated value of 'zeta' for Group 1: 0.22454 Updated value of 'sigma2' for Group 1: 0.508693 Updated value of 'sig2e': 0.045310 --------------------------------------------------------- SP_MMV_FXN: Completed 22 iterations Total elapsed time: 0.423261 s Time-averaged residual energy: 2.244928 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.064830 Updated value of 'alpha' for Group 1: 0.384863 Updated value of 'zeta' for Group 1: 0.22454 Updated value of 'sigma2' for Group 1: 0.492925 Updated value of 'sig2e': 0.044556 --------------------------------------------------------- SP_MMV_FXN: Completed 23 iterations Total elapsed time: 0.436464 s Time-averaged residual energy: 2.169290 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.064830 Updated value of 'alpha' for Group 1: 0.384863 Updated value of 'zeta' for Group 1: 0.23186 Updated value of 'sigma2' for Group 1: 0.477453 Updated value of 'sig2e': 0.043615 --------------------------------------------------------- SP_MMV_FXN: Completed 24 iterations Total elapsed time: 0.449617 s Time-averaged residual energy: 2.085301 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.065993 Updated value of 'alpha' for Group 1: 0.375939 Updated value of 'zeta' for Group 1: 0.23186 Updated value of 'sigma2' for Group 1: 0.463683 Updated value of 'sig2e': 0.042722 --------------------------------------------------------- SP_MMV_FXN: Completed 25 iterations Total elapsed time: 0.462993 s Time-averaged residual energy: 2.008562 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.065993 Updated value of 'alpha' for Group 1: 0.375939 Updated value of 'zeta' for Group 1: 0.23847 Updated value of 'sigma2' for Group 1: 0.450916 Updated value of 'sig2e': 0.041726 --------------------------------------------------------- SP_MMV_FXN: Completed 26 iterations Total elapsed time: 0.477072 s Time-averaged residual energy: 1.912886 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.066838 Updated value of 'alpha' for Group 1: 0.367724 Updated value of 'zeta' for Group 1: 0.23847 Updated value of 'sigma2' for Group 1: 0.438721 Updated value of 'sig2e': 0.040134 --------------------------------------------------------- SP_MMV_FXN: Completed 27 iterations Total elapsed time: 0.491359 s Time-averaged residual energy: 1.866082 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.066838 Updated value of 'alpha' for Group 1: 0.367724 Updated value of 'zeta' for Group 1: 0.24502 Updated value of 'sigma2' for Group 1: 0.427930 Updated value of 'sig2e': 0.038075 --------------------------------------------------------- SP_MMV_FXN: Completed 28 iterations Total elapsed time: 0.505762 s Time-averaged residual energy: 1.822188 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.066683 Updated value of 'alpha' for Group 1: 0.360134 Updated value of 'zeta' for Group 1: 0.24502 Updated value of 'sigma2' for Group 1: 0.417050 Updated value of 'sig2e': 0.036023 --------------------------------------------------------- SP_MMV_FXN: Completed 29 iterations Total elapsed time: 0.520086 s Time-averaged residual energy: 1.733974 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.066683 Updated value of 'alpha' for Group 1: 0.360134 Updated value of 'zeta' for Group 1: 0.25102 Updated value of 'sigma2' for Group 1: 0.407966 Updated value of 'sig2e': 0.034221 --------------------------------------------------------- SP_MMV_FXN: Completed 30 iterations Total elapsed time: 0.533831 s Time-averaged residual energy: 1.590650 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.068066 Updated value of 'alpha' for Group 1: 0.353297 Updated value of 'zeta' for Group 1: 0.25102 Updated value of 'sigma2' for Group 1: 0.398465 Updated value of 'sig2e': 0.032787 --------------------------------------------------------- SP_MMV_FXN: Completed 31 iterations Total elapsed time: 0.547430 s Time-averaged residual energy: 1.487443 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.068066 Updated value of 'alpha' for Group 1: 0.353297 Updated value of 'zeta' for Group 1: 0.2579 Updated value of 'sigma2' for Group 1: 0.392297 Updated value of 'sig2e': 0.031253 --------------------------------------------------------- SP_MMV_FXN: Completed 32 iterations Total elapsed time: 0.559938 s Time-averaged residual energy: 1.428560 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.068558 Updated value of 'alpha' for Group 1: 0.347332 Updated value of 'zeta' for Group 1: 0.2579 Updated value of 'sigma2' for Group 1: 0.384200 Updated value of 'sig2e': 0.030036 --------------------------------------------------------- SP_MMV_FXN: Completed 33 iterations Total elapsed time: 0.572346 s Time-averaged residual energy: 1.370005 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.068558 Updated value of 'alpha' for Group 1: 0.347332 Updated value of 'zeta' for Group 1: 0.26483 Updated value of 'sigma2' for Group 1: 0.379887 Updated value of 'sig2e': 0.029278 --------------------------------------------------------- SP_MMV_FXN: Completed 34 iterations Total elapsed time: 0.584883 s Time-averaged residual energy: 1.322940 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.069036 Updated value of 'alpha' for Group 1: 0.341981 Updated value of 'zeta' for Group 1: 0.26483 Updated value of 'sigma2' for Group 1: 0.372757 Updated value of 'sig2e': 0.028695 --------------------------------------------------------- SP_MMV_FXN: Completed 35 iterations Total elapsed time: 0.597590 s Time-averaged residual energy: 1.282074 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.069036 Updated value of 'alpha' for Group 1: 0.341981 Updated value of 'zeta' for Group 1: 0.27159 Updated value of 'sigma2' for Group 1: 0.370081 Updated value of 'sig2e': 0.028143 --------------------------------------------------------- SP_MMV_FXN: Completed 36 iterations Total elapsed time: 0.610771 s Time-averaged residual energy: 1.257515 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.068963 Updated value of 'alpha' for Group 1: 0.337243 Updated value of 'zeta' for Group 1: 0.27159 Updated value of 'sigma2' for Group 1: 0.363852 Updated value of 'sig2e': 0.027365 --------------------------------------------------------- SP_MMV_FXN: Completed 37 iterations Total elapsed time: 0.623195 s Time-averaged residual energy: 1.223247 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.068963 Updated value of 'alpha' for Group 1: 0.337243 Updated value of 'zeta' for Group 1: 0.27787 Updated value of 'sigma2' for Group 1: 0.362583 Updated value of 'sig2e': 0.026613 --------------------------------------------------------- SP_MMV_FXN: Completed 38 iterations Total elapsed time: 0.635707 s Time-averaged residual energy: 1.194271 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.069079 Updated value of 'alpha' for Group 1: 0.333010 Updated value of 'zeta' for Group 1: 0.27787 Updated value of 'sigma2' for Group 1: 0.357067 Updated value of 'sig2e': 0.025851 --------------------------------------------------------- SP_MMV_FXN: Completed 39 iterations Total elapsed time: 0.648179 s Time-averaged residual energy: 1.165853 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.069079 Updated value of 'alpha' for Group 1: 0.333010 Updated value of 'zeta' for Group 1: 0.28388 Updated value of 'sigma2' for Group 1: 0.356760 Updated value of 'sig2e': 0.025219 --------------------------------------------------------- SP_MMV_FXN: Completed 40 iterations Total elapsed time: 0.660738 s Time-averaged residual energy: 1.147213 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.069520 Updated value of 'alpha' for Group 1: 0.329081 Updated value of 'zeta' for Group 1: 0.28388 Updated value of 'sigma2' for Group 1: 0.351672 Updated value of 'sig2e': 0.024554 --------------------------------------------------------- SP_MMV_FXN: Completed 41 iterations Total elapsed time: 0.673149 s Time-averaged residual energy: 1.124605 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.069520 Updated value of 'alpha' for Group 1: 0.329081 Updated value of 'zeta' for Group 1: 0.28935 Updated value of 'sigma2' for Group 1: 0.351771 Updated value of 'sig2e': 0.023957 --------------------------------------------------------- SP_MMV_FXN: Completed 42 iterations Total elapsed time: 0.685782 s Time-averaged residual energy: 1.106094 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.070019 Updated value of 'alpha' for Group 1: 0.325342 Updated value of 'zeta' for Group 1: 0.28935 Updated value of 'sigma2' for Group 1: 0.346950 Updated value of 'sig2e': 0.023366 --------------------------------------------------------- SP_MMV_FXN: Completed 43 iterations Total elapsed time: 0.698219 s Time-averaged residual energy: 1.081729 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.070019 Updated value of 'alpha' for Group 1: 0.325342 Updated value of 'zeta' for Group 1: 0.29416 Updated value of 'sigma2' for Group 1: 0.347349 Updated value of 'sig2e': 0.022900 --------------------------------------------------------- SP_MMV_FXN: Completed 44 iterations Total elapsed time: 0.710791 s Time-averaged residual energy: 1.063222 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.070472 Updated value of 'alpha' for Group 1: 0.321801 Updated value of 'zeta' for Group 1: 0.29416 Updated value of 'sigma2' for Group 1: 0.342798 Updated value of 'sig2e': 0.022485 --------------------------------------------------------- SP_MMV_FXN: Completed 45 iterations Total elapsed time: 0.723225 s Time-averaged residual energy: 1.041249 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.070472 Updated value of 'alpha' for Group 1: 0.321801 Updated value of 'zeta' for Group 1: 0.29843 Updated value of 'sigma2' for Group 1: 0.343561 Updated value of 'sig2e': 0.022186 --------------------------------------------------------- SP_MMV_FXN: Completed 46 iterations Total elapsed time: 0.735748 s Time-averaged residual energy: 1.026957 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.070844 Updated value of 'alpha' for Group 1: 0.318471 Updated value of 'zeta' for Group 1: 0.29843 Updated value of 'sigma2' for Group 1: 0.339290 Updated value of 'sig2e': 0.021913 --------------------------------------------------------- SP_MMV_FXN: Completed 47 iterations Total elapsed time: 0.748191 s Time-averaged residual energy: 1.009478 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.070844 Updated value of 'alpha' for Group 1: 0.318471 Updated value of 'zeta' for Group 1: 0.30228 Updated value of 'sigma2' for Group 1: 0.340435 Updated value of 'sig2e': 0.021719 --------------------------------------------------------- SP_MMV_FXN: Completed 48 iterations Total elapsed time: 0.760732 s Time-averaged residual energy: 0.999727 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.071131 Updated value of 'alpha' for Group 1: 0.315351 Updated value of 'zeta' for Group 1: 0.30228 Updated value of 'sigma2' for Group 1: 0.336436 Updated value of 'sig2e': 0.021525 --------------------------------------------------------- SP_MMV_FXN: Completed 49 iterations Total elapsed time: 0.773032 s Time-averaged residual energy: 0.985724 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.071131 Updated value of 'alpha' for Group 1: 0.315351 Updated value of 'zeta' for Group 1: 0.30579 Updated value of 'sigma2' for Group 1: 0.337936 Updated value of 'sig2e': 0.021388 --------------------------------------------------------- SP_MMV_FXN: Completed 50 iterations Total elapsed time: 0.785599 s Time-averaged residual energy: 0.978986 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.071353 Updated value of 'alpha' for Group 1: 0.312428 Updated value of 'zeta' for Group 1: 0.30579 Updated value of 'sigma2' for Group 1: 0.334185 Updated value of 'sig2e': 0.021239 --------------------------------------------------------- SP_MMV_FXN: Completed 51 iterations Total elapsed time: 0.798036 s Time-averaged residual energy: 0.966861 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.071353 Updated value of 'alpha' for Group 1: 0.312428 Updated value of 'zeta' for Group 1: 0.30898 Updated value of 'sigma2' for Group 1: 0.335997 Updated value of 'sig2e': 0.021135 --------------------------------------------------------- SP_MMV_FXN: Completed 52 iterations Total elapsed time: 0.810593 s Time-averaged residual energy: 0.961591 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.071536 Updated value of 'alpha' for Group 1: 0.309684 Updated value of 'zeta' for Group 1: 0.30898 Updated value of 'sigma2' for Group 1: 0.332471 Updated value of 'sig2e': 0.021016 --------------------------------------------------------- SP_MMV_FXN: Completed 53 iterations Total elapsed time: 0.823067 s Time-averaged residual energy: 0.950075 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.071536 Updated value of 'alpha' for Group 1: 0.309684 Updated value of 'zeta' for Group 1: 0.31192 Updated value of 'sigma2' for Group 1: 0.334563 Updated value of 'sig2e': 0.020937 --------------------------------------------------------- SP_MMV_FXN: Completed 54 iterations Total elapsed time: 0.835608 s Time-averaged residual energy: 0.945038 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.071697 Updated value of 'alpha' for Group 1: 0.307108 Updated value of 'zeta' for Group 1: 0.31192 Updated value of 'sigma2' for Group 1: 0.331242 Updated value of 'sig2e': 0.020841 --------------------------------------------------------- SP_MMV_FXN: Completed 55 iterations Total elapsed time: 0.848022 s Time-averaged residual energy: 0.933030 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.071697 Updated value of 'alpha' for Group 1: 0.307108 Updated value of 'zeta' for Group 1: 0.3147 Updated value of 'sigma2' for Group 1: 0.333603 Updated value of 'sig2e': 0.020781 --------------------------------------------------------- SP_MMV_FXN: Completed 56 iterations Total elapsed time: 0.860626 s Time-averaged residual energy: 0.926962 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.071853 Updated value of 'alpha' for Group 1: 0.304696 Updated value of 'zeta' for Group 1: 0.3147 Updated value of 'sigma2' for Group 1: 0.330482 Updated value of 'sig2e': 0.020705 --------------------------------------------------------- SP_MMV_FXN: Completed 57 iterations Total elapsed time: 0.873088 s Time-averaged residual energy: 0.913060 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.071853 Updated value of 'alpha' for Group 1: 0.304696 Updated value of 'zeta' for Group 1: 0.31742 Updated value of 'sigma2' for Group 1: 0.333129 Updated value of 'sig2e': 0.020658 --------------------------------------------------------- SP_MMV_FXN: Completed 58 iterations Total elapsed time: 0.885657 s Time-averaged residual energy: 0.904160 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.07 Updated value of 'alpha' for Group 1: 0.302458 Updated value of 'zeta' for Group 1: 0.31742 Updated value of 'sigma2' for Group 1: 0.330217 Updated value of 'sig2e': 0.020590 --------------------------------------------------------- SP_MMV_FXN: Completed 59 iterations Total elapsed time: 0.898092 s Time-averaged residual energy: 0.886368 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.07 Updated value of 'alpha' for Group 1: 0.302458 Updated value of 'zeta' for Group 1: 0.32029 Updated value of 'sigma2' for Group 1: 0.333211 Updated value of 'sig2e': 0.020534 --------------------------------------------------------- SP_MMV_FXN: Completed 60 iterations Total elapsed time: 0.910646 s Time-averaged residual energy: 0.873161 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.072087 Updated value of 'alpha' for Group 1: 0.300418 Updated value of 'zeta' for Group 1: 0.32029 Updated value of 'sigma2' for Group 1: 0.330540 Updated value of 'sig2e': 0.020422 --------------------------------------------------------- SP_MMV_FXN: Completed 61 iterations Total elapsed time: 0.923091 s Time-averaged residual energy: 0.854081 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.072087 Updated value of 'alpha' for Group 1: 0.300418 Updated value of 'zeta' for Group 1: 0.32351 Updated value of 'sigma2' for Group 1: 0.333979 Updated value of 'sig2e': 0.020224 --------------------------------------------------------- SP_MMV_FXN: Completed 62 iterations Total elapsed time: 0.936200 s Time-averaged residual energy: 0.838597 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.071465 Updated value of 'alpha' for Group 1: 0.298595 Updated value of 'zeta' for Group 1: 0.32351 Updated value of 'sigma2' for Group 1: 0.331574 Updated value of 'sig2e': 0.019783 --------------------------------------------------------- SP_MMV_FXN: Completed 63 iterations Total elapsed time: 0.949490 s Time-averaged residual energy: 0.794747 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.071465 Updated value of 'alpha' for Group 1: 0.298595 Updated value of 'zeta' for Group 1: 0.32773 Updated value of 'sigma2' for Group 1: 0.335717 Updated value of 'sig2e': 0.019162 --------------------------------------------------------- SP_MMV_FXN: Completed 64 iterations Total elapsed time: 0.963824 s Time-averaged residual energy: 0.729865 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.069404 Updated value of 'alpha' for Group 1: 0.297168 Updated value of 'zeta' for Group 1: 0.32773 Updated value of 'sigma2' for Group 1: 0.333813 Updated value of 'sig2e': 0.018059 --------------------------------------------------------- SP_MMV_FXN: Completed 65 iterations Total elapsed time: 0.978542 s Time-averaged residual energy: 0.645099 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.069404 Updated value of 'alpha' for Group 1: 0.297168 Updated value of 'zeta' for Group 1: 0.33478 Updated value of 'sigma2' for Group 1: 0.340463 Updated value of 'sig2e': 0.016139 --------------------------------------------------------- SP_MMV_FXN: Completed 66 iterations Total elapsed time: 0.993424 s Time-averaged residual energy: 0.515320 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.067385 Updated value of 'alpha' for Group 1: 0.296802 Updated value of 'zeta' for Group 1: 0.33478 Updated value of 'sigma2' for Group 1: 0.339964 Updated value of 'sig2e': 0.013314 --------------------------------------------------------- SP_MMV_FXN: Completed 67 iterations Total elapsed time: 1.005852 s Time-averaged residual energy: 0.442852 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.067385 Updated value of 'alpha' for Group 1: 0.296802 Updated value of 'zeta' for Group 1: 0.34855 Updated value of 'sigma2' for Group 1: 0.350472 Updated value of 'sig2e': 0.011519 --------------------------------------------------------- SP_MMV_FXN: Completed 68 iterations Total elapsed time: 1.018401 s Time-averaged residual energy: 0.396004 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.067883 Updated value of 'alpha' for Group 1: 0.297143 Updated value of 'zeta' for Group 1: 0.34855 Updated value of 'sigma2' for Group 1: 0.350949 Updated value of 'sig2e': 0.010267 --------------------------------------------------------- SP_MMV_FXN: Completed 69 iterations Total elapsed time: 1.030839 s Time-averaged residual energy: 0.357496 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.067883 Updated value of 'alpha' for Group 1: 0.297143 Updated value of 'zeta' for Group 1: 0.36459 Updated value of 'sigma2' for Group 1: 0.362317 Updated value of 'sig2e': 0.009395 --------------------------------------------------------- SP_MMV_FXN: Completed 70 iterations Total elapsed time: 1.043474 s Time-averaged residual energy: 0.331105 --------------------------------------------------------- Updated value of 'lambda' for Group 1: 0.068498 Updated value of 'alpha' for Group 1: 0.297507 Updated value of 'zeta' for Group 1: 0.36459 Updated value of 'sigma2' for Group 1: 0.362844 Updated value of 'sig2e': 0.008762 --------------------------------------------------------- sp_mmv_fxn: Max # of smoothing iterations reached
And let's compute our performance metrics on the recovery we obtained using this schedule, and plot the recovery:
s_hat = (lambda_hat > 1/2); TNMSE = sum(sum(([x_true{:}]-[x_hat{:}]).^2, 1)./sum([x_true{:}].^2, 1))/T; NSER = nser(support, find(s_hat == 1)); fprintf('TNMSE: %g dB\n', 10*log10(TNMSE)); fprintf('NSER: %g\n', NSER); figure(1); subplot(121); imagesc([x_true{:}]); colorbar xlabel('Timestep (t)'); ylabel('Index [n]'); title('True signal') subplot(122); imagesc([x_hat{:}]); colorbar xlabel('Timestep (t)'); ylabel('Index [n]'); title('AMP-MMV recovery')
TNMSE: -24.5377 dB NSER: 0.0140845
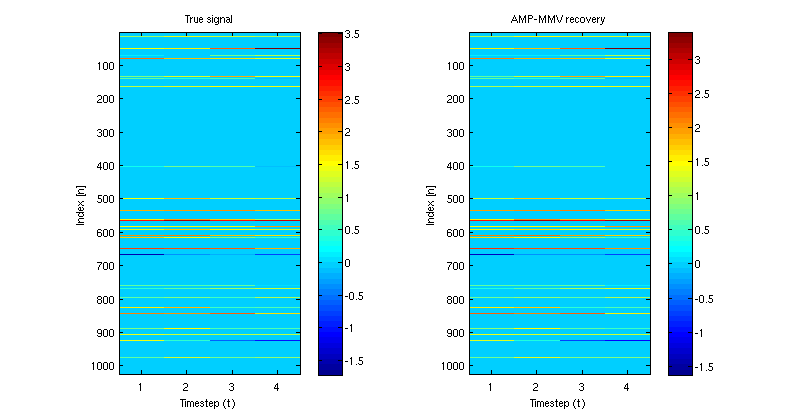
Now let's run the support-aware Kalman smoother so that we can see how well AMP-MMV performed compared to this genie-aided bound. The function that we will call is GENIE_MULTI_FRAME_FXN. In order to obtain a meaningful bound, we will provide the function with the true underlying model parameters. Let's construct this ModelParams object by using the constructor that simply copies over the information from our SigGenParams object, SigGenObj:
SKSParams = ModelParams(SigGenObj, sig2e); SKSParams.print();
**************************************** Signal Model Parameters **************************************** Activity probability (lambda): 0.0625 Active mean (zeta): 1 Active variance (sigma2): 1 Innovation rate (alpha): 0.1 AWGN variance (sig2e): 0.0026064
We will also create a custom Options object that tells GENIE_MULTI_FRAME_FXN not to use the AMP algorithm to perform message passing within each frame, but rather use the slower (but theoretically grounded) exact belief propagation algorithm.
SKSRunOpt = Options('smooth_iters', 200, 'alg', 'BP'); SKSRunOpt.print();
*********************************** AMP-MMV Runtime Options *********************************** Max. smoothing iterations: 200 Min. smoothing iterations: 5 Max. AMP/BP iterations: 15 Intra-frame algorithm: Gaussian BP EM parameter learning: Yes Update groups: [Default] Verbosity: Silent Warm-Start: No epsilon: 1e-06 f-to-theta approx (tau): Taylor series approx
Now, recalling that support is the variable that contains the true signal support, let's run the SKS:
x_sks = genie_multi_frame_fxn(y, A, support, SKSParams, SKSRunOpt);
Now let's see how AMP-MMV stacked up against the SKS by calculating the TNMSE of the SKS recovery (note that the NSER for the SKS will always be zero)
TNMSE = sum(sum(([x_true{:}]-[x_sks{:}]).^2, 1)./sum([x_true{:}].^2, 1))/T; fprintf('TNMSE: %g dB\n', 10*log10(TNMSE)); figure(1); subplot(121); imagesc([x_true{:}]); colorbar xlabel('Timestep (t)'); ylabel('Index [n]'); title('True signal') subplot(122); imagesc([x_sks{:}]); colorbar xlabel('Timestep (t)'); ylabel('Index [n]'); title('SKS recovery')
TNMSE: -25.1342 dB
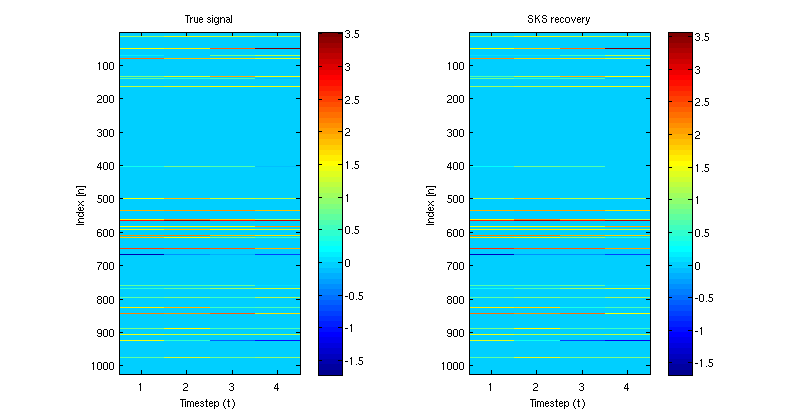